Linking apps together with App Links
When developing apps for mobile phones you want to offer your users the best user experience. Sometimes this includes showing information outside of your app, in another app. Up until recently, there was no real good way to do this. Luckily, now there is a new initiative App Links that provides an open source and cross-platform solution for app-to-app linking. The initiative is supported by many mobile app developers, like Dropbox, Facebook, Spotify and Pinterest. In this post I will show you an example how to link between two Android applications using the open source implementation for Android Bolts. However, the same principles apply when you want to link between two iOS applications.
App Links
Creating an App Link can be done by simply adding a few metadata properties in the head tag of your HTML page. This metadata can be used to (deep-)link into your app or another app. If the user doesn’t have your app installed, the metadata can also be used to send the user to the correct app store to download the app. Another option that App Links provide is to take the user directly to the web view of the content.
One library that simplify the implementation of App Links is Bolts. Bolts provides helper methods to receive or navigate to an App Link.
In my example I have two apps, one with basic information about patients and one to register how long a treatment of this patient took (always one hour in my app). I have also two HTML pages which are used to get the metadata from that contains the information of which app needs to be opened.
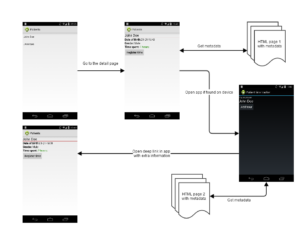
HTML page 1 containing metadata to open the time tracker app:
</p> <p></pre><br> <pre><html><br> <head><br> <meta property="al:android:package" content="nl.trifork.patienttimetracker" /><br> <meta property="al:android:app_name" content="Patient time tracker" /><br> </head><br> <body>Patient time tracker</body><br> </html><br>
Patients app
In the patients app I created the functionality to open the time tracker app. It will collect the metadata properties from the url of HTML page 1. The metadata contains the package name of the time tracker app, which will be used to create an Intent.
To check if the Intent could be opened by any of my installed apps I call the method PackageManager.resolveActivity(). If this method returns any ResolveInfo then it has found an app that could open your link. In my case it will of course find my time tracker app :). The time tracker will open the main activity as we didn’t add the metadata property for a classname.
In order to send some data from the patients app to the time tracker app, we need to create a Bundle and add it to the Intent as an Extra named al_applink_data. Once we have done that we can start an Activity for our constructed Intent.
<br> private void startActivityForAppLink(final String name,<br> final String gender,<br> final String dob,<br> final int time) {<br> new WebViewAppLinkResolver(this)<br> .getAppLinkFromUrlInBackground(Uri.parse(TIME_REGISTRATION_URL))<br> .continueWith(<br> new Continuation<AppLink, AppLinkNavigation.NavigationResult>() {<br> @Override<br> public AppLinkNavigation.NavigationResult then(<br> Task<AppLink> task) {<br> AppLink link = task.getResult();</p> <p> Intent intent = new Intent(Intent.ACTION_VIEW);<br> AppLink.Target target = link.getTargets().get(0);<br> intent.setPackage(target.getPackageName());<br> intent.setData(link.getSourceUrl());</p> <p> ResolveInfo resolveInfo = getPackageManager()<br> .resolveActivity(intent,<br> PackageManager.MATCH_DEFAULT_ONLY);<br> if (resolveInfo != null) {<br> Bundle extras = new Bundle();<br> extras.putString("target_url", TIME_REGISTRATION_URL);<br> extras.putString("name", name);<br> extras.putString("gender", gender);<br> extras.putString("dob", dob);<br> extras.putInt("time", time);</p> <p> intent.putExtra("al_applink_data", extras);<br> startActivity(intent);<br> }<br> return null;<br> }<br> }<br> );<br> }<br>
HTML page 2 containing metadata to open the Patients app to deep-link to the information of a patient:
<br> <html><br> <head><br> <meta property="al:android:package"<br> content="nl.trifork.applinks" /><br> <meta property="al:android:app_name"<br> content="Patient info" /><br> <meta property="al:android:class"<br> content="nl.trifork.applinks.PatientItemActivity" /><br> </head><br> <body></body><br> </html><br>
Time tracker app
This app is created to receive incoming App Links. To make the app listen to Intents you need to specify an Intent filter to the activity that handles the incoming App Links:
<br> <intent-filter><br> <action android:name="android.intent.action.VIEW" /><br> <category android:name="android.intent.category.DEFAULT" /><br> <data android:scheme="http" /><br> </intent-filter><br>
To get the incoming App Link data you can call AppLinks.getAppLinkData(getIntent())
. This will give you the information as a Bundle, which contains all the data we passed in from the Patients app.
<br> Bundle appLinkData = AppLinks.getAppLinkData(intent);<br> if (appLinkData != null) {<br> final String patientName = appLinkData.getString("name");<br> TextView patientNameValueView = (TextView)<br> findViewById(R.id.patientNameValueView);<br> patientNameValueView.setText(patientName);</p> <p> final String gender = appLinkData.getString("gender");<br> final String dob = appLinkData.getString("dob");<br> final int time = appLinkData.getInt("time");<br> }<br>
Deep linking
When I have registered some time for a patient I want to update my view in the Patients app. The patient detail view is reachable from a list of patients and not directly in the app. I have configured an Intent filter on the detail view, so we are able to receive App Links.
I call the url of HTML page 2 which contains the property of the classname as metadata. With this metadata we can construct a more specific Intent the same way we did earlier. This way the package manager can resolve the corresponding view and we can deep link into our app with some extra data. On the flow image you can see that the registered time has been upped by one hour.
Other features of App Links
In my example I have used a website to get the metadata from, but it is also possible to publish your links via another service like Parse or Facebook. Also my apps are not available in the app store, but as said before App Links provides you with the option to send the user to the store when an app is not installed on your device.
You can download the full source code here: source code
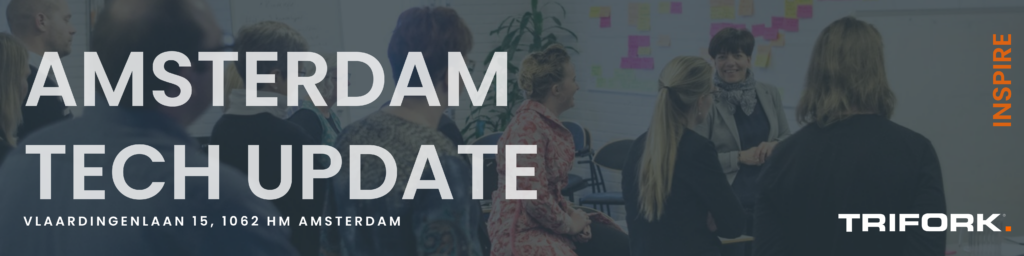