Refactoring from Elasticsearch version 1 with Java Transport client to version 6 with High Level REST client
Every long running project accrues technical debt. It may be that the requirements today have evolved in a different direction from what was foreseen when the project was designed, or it may be that difficult infrastructure tasks have been put off in favor of new functionality. From time to time, you need to refactor your code to clean up this technical debt. I recently finished such a refactoring task for a customer, so in the category ‘from the trenches’, I would like to share the story here.
Elasticsearch exposes both a REST interface and the internal Java API, via the binary transport client, for connecting with the search engine. Just over a year ago, Elastic announced to the world that it plans to deprecate the transport client in favor of the high level REST client, “as soon as the REST client is feature complete and is mature enough to replace the Java API entirely”. The reasons for this are clearly explained in Luca Cavanna’s blogpost, but the most important disadvantage is that using the transport client, you introduce a tight coupling between your application and the exact major and minor release of your ES cluster. As long as Elasticsearch exposes its internal API, it has to worry about breaking thousands of applications all over the world that depend on it.
The “as soon as…” timetable sounds somewhat vague and long term, but there may be good reasons to migrate your search functionality now, rather than later. In the case of our customer, their reason is wanting to use the AWS Elasticsearch service. The entire codebase is already running in AWS, and for the past few years they have been managing their own Elasticsearch cluster running in EC2 instances. This turns out to be labor intensive when updates have to be applied to these VMs. It would be easier and probably cheaper to let Amazon manage the cluster. As the AWS Elasticsearch service only exposes the REST API, the dependence on the transport protocol will have to be removed.
Action plan
The starting situation was a dependency on Elasticsearch 1.4.5, using the Java API. The goal was the most recent Elasticsearch version available in the Amazon Elasticsearch Service, which at the time was 6.0.2, using the REST API.
In order to reduce the complexity of the refactoring operation, we decided early on, to reindex the data, rather than trying to convert the indices. Every Elasticsearch release comes with a handy list of breaking changes. Looking through this list, we tried to make a list of breaking changes that would likely affect the search implementation of our customer. There are more potential breaking changes than listed here, but these are the ones that an initial investigation suggested might have an impact:
1.x – 2.x:
- Facets replaced by aggregations
- Field names can’t contain dots
2.x – 5.x:
- Suggest API refactored
- TransportClient refactored and moved to a separate dependency
- String type split into ‘text’ and ‘keyword’
5.x – 6.0:
- Support for indices with multiple mapping types dropped
The plan was first to convert the existing code to work with ES 6, and only then migrate from the transport client to the High Level REST client.
Implementation
The entire search functionality, originally written by our former colleague Frans Flippo, was exhaustively covered by unit- and integration tests, so the first step was to update the maven dependency to the current version, run the tests, and see what broke. First there were compilation errors that were easily fixed. Some examples:
Replace FilterBuilder with QueryBuilder, RangeFilterBuilder with RangeQueryBuilder, TermsFilterBuilder with TermsQueryBuilder, PercolateRequestBuilder with PercolateQueryBuilder etc, switch to HighlightBuilder for highlighters, replace ‘fields’ with ‘storedFields’. The count API was removed in version 5.5, and its use had to be replaced by executing a search with size 0. Facets had already been replaced by aggregations by our colleague Attila Houtkooper, so we didn’t have to worry about that.
In ES 5, the suggest API was removed, and became part of the search API. This turned out not to have an impact on our project, because the original developer of the search functionality implemented a custom suggestions service based on aggregation queries. It looks like he wanted the suggestions to be ordered by the number of occurrences in a ‘bucket’, which couldn’t be implemented using the suggest API at the time. We decided that refactoring this to use Elasticsearch suggesters would be new functionality, and outside the scope of this upgrade, so we would continue to use aggregations for now.
Some updates were required to the index mappings. The most obvious one was replacing ‘string’ with either ‘text’ or ‘keyword’. Analyzer became search_analyzer, while index_analyzer became analyzer.
Syntax ES 1:
<br> "fields": {<br> "analyzed": {<br> "type": "string",<br> "analyzer" : "dutch",<br> "index_analyzer": "default_min_word_length_2"<br> },<br> "not_analyzed": {<br> "type": "string",<br> "index": "not_analyzed"<br> }<br> }<br>
Syntax ES 6:
<br> "fields": {<br> "analyzed": {<br> "type": "text",<br> "search_analyzer": "dutch",<br> "analyzer": "default_min_word_length_2"<br> },<br> "not_analyzed": {<br> "type": "keyword",<br> "index": true<br> }<br> }<br>
Document id’s were associated with a path:
<br> "_id": {<br> "path": "id"<br> },<br>
The _id field is no longer configurable, so in order to have document ids in Elasticsearch match ids in the database, the id has to be set explicitly, or Elasticsearch will generate a random one.
All in all, it was roughly a day of work to get the project to compile and ready to run the unit tests. All of them were red.
Running Elasticsearch integration tests
The integration tests depended on a framework that spun up an embedded Elasticsearch node, to run the tests against. This mechanism is no longer supported. Though with some effort it is still possible to get this to work, the main point of the operation was to move the search implementation back into ‘supported’ territory, so we decided to abandon this approach.
First we tried out the integration test framework offered by Elasticsearch: ESIntegTestCase. Unfortunately, this framework turns out to be highly opinionated. It wants the tests to run under the RandomizedRunner, which is also used to test Lucene. In order to work with the ESIntegTestCase, we would have had to partially rewrite most of the existing integration tests. Ideally, you can rely on the unit tests to prove that your refactoring preserved the expected functionality. If you have to change the tests, you run the risk that you end up ‘fixing’ a test to make it green. We decided to go with the Elasticsearch maven plugin instead, which required no code changes to the tests other than configuring the ES client.
<br> <plugin><br> <groupId>com.github.alexcojocaru</groupId><br> <artifactId>elasticsearch-maven-plugin</artifactId><br> <version>6.0</version> <!--Plugin version--><br> <configuration><br> <clusterName>testCluster</clusterName><br> <transportPort>9500</transportPort><br> <httpPort>9400</httpPort><br> <version>6.0.2</version> <!--Elasticsearch version--><br> <timeout>20</timeout><br> </configuration><br> <executions><br> <execution><br> <id>start-elasticsearch</id><br> <phase>pre-integration-test</phase><br> <goals><br> <goal>runforked</goal><br> </goals><br> </execution><br> <execution><br> <id>stop-elasticsearch</id><br> <phase>post-integration-test</phase><br> <goals><br> <goal>stop</goal><br> </goals><br> </execution><br> </executions><br> </plugin><br>
That’s all there is to it. With this configuration, when maven enters the pre-integration test phase, the plugin starts a single node elasticsearch cluster of version 6.0.2 in a new process, listening to ports 9500 and 9400, runs the tests, and then stops the cluster and cleans up. The plugin allows a more fine grained configuration of the cluster, including ES plugins, but we were trying to replace a simple embedded ES node, so this was unnecessary. Now all the tests were yellow: the project compiles, tests run and assertions fail.
Fixing the pitfalls
Some search results were missing data. In version 1.x, fields added to your query were retrieved from the _source field, and returned in your search result. Where we replaced these with storedFields, the fields in question had to be explicitly marked as stored fields in the mapping. Fields that are not stored, are included in your search, but not returned in the search result. This can be useful in queries where you want to retrieve just a few fields from a large document.
Some aggregations were failing with the message ‘Fielddata is disabled on text fields by default’. In ES 1, there were ‘string’ fields, not ‘text’ and ‘keyword’ fields. By default, operations like sorting and aggregations are not allowed on ‘text’ fields, unless you explicitly mark the field as such, with “fielddata”:true. This is generally not a good idea on analyzed fields, as it can cause a substantial performance hit, and may return results you were probably not expecting. We decided to use “copy_to” to make ‘keyword’ type copies of the fields in question to run the aggregations on.
It seems that ES 1.4 supported the java regex engine, while ES 6.x uses a different one, which doesn’t support boundary matchers such as \b. Luckily there was a workaround, which should work in most cases.
In the REST API, a query field can be boosted with a caret: “fields”:[ “title^5”]. In this search implementation based on the transport client, this was implemented by appending ^ and the boost to the field name: “title^”+titleBoost. In ES 6, this approach no longer seemed to work. There was a clear difference in results between a query through the Java API, and executing the exact same query, via the toString() method of the QueryBuilder, using the REST API. The correct approach is myQueryStringQueryBuilder.field(fieldName, boost). Because the query looked fine in the console, and worked fine when copied and run against the REST API, this pitfall was not immediately obvious.
Differences in search results
A fair number of the tests failed because the order of search results by relevance had changed between ES 1 and 6. From the way the tests were written, we got the impression that in ES 1, documents with the exact same score were returned in the order in which they were indexed, while in ES 6, this doesn’t seem to be the case. We could have made the tests ‘green’, by adding an additional sort by id after the _score, which would make the test perform consistently over our tiny artificial test data set, but this didn’t ‘feel’ right. We didn’t find an explicit mention of this behavior in the documentation, or any change over ES versions, and there wasn’t a good use case for it. The numerical value of the document id has no special relevance to the users. In real world data, search results with the exact same _score would probably be exactly equally relevant, and the customer agreed with us not to second guess Elasticsearch and Lucene on the matter of search result relevance.
A more interesting issue was a test where documents that looked more relevant to us, were now getting a lower score than seemingly less relevant ones. The tiny data set for these tests contained a large number of mentions of the search term in the queries. Somehow, documents that contained an additional, boosted, field that matched the search term, were scoring lower than an otherwise identical document where one of those fields was left blank. The weight of a search term is the product of a function that combines the term frequency, the inverse document frequency and the boost on a field. Artificially packing a document with more mentions of the search term was actually making a match on this search term less relevant to Lucene. We could have spent considerable time tweaking the queries to make documents in our very artificial test data set equally relevant to ES 6 as they were to ES 1, but the customer agreed with us that this wasn’t the way to go. Considerable effort in the development of search engines goes into making search results more relevant. It seemed like a good idea to trust the experts at Elastic and Lucene when it comes to search result relevance, so the customer agreed we shouldn’t spend a lot of time trying to replicate the behavior of a more primitive version of the search engine, fine tuned to a tiny, artificial test data set. All tests were now green. On to the next stage.
Migrating to the High Level REST Client
In order to work with the Amazon Elasticsearch Service, we had to remove the dependence on the transport client. In the long term, this is a good idea anyway, as Elastic intends to deprecate and ultimately remove this client. In order to facilitate the move away from the transport client, ES has been working on the so called High Level REST Client. This client uses the low level REST client to send requests, but accepts the existing query builders from the Java API, and returns the same response objects. At least in theory, this should allow you to move your search functionality to the new client with minimal code changes. When it came to searching, practice matched this theory quite nicely. Here is a pseudocode representation of the syntax using the transport client:
<br> // Query<br> SearchRequestBuilder searchRequestBuilder = client.prepareSearch(indexName);<br> searchRequestBuilder.setTypes(objectType);<br> searchRequestBuilder.setQuery(buildMainQuery(formObject));</p> <p>// Paging<br> searchRequestBuilder.setFrom(maxSize * formObject.getPage());<br> searchRequestBuilder.setSize(maxSize);</p> <p>// Fields to return<br> for (String field : formObject.getRequestedFields()) {<br> searchRequestBuilder.addStoredField(field);<br> }</p> <p>// Filters<br> searchRequestBuilder.setPostFilter(buildFilterQuery(formObject));</p> <p>// Sorting<br> searchRequestBuilder.addSort(formObject.getSortField(), toSortOrder(formObject.getSortOrder()));</p> <p>(...)</p> <p>SearchResponse searchResponse = searchRequestBuilder.execute().actionGet();<br>
And here is the syntax using the High Level REST Client
<br> // Query<br> SearchSourceBuilder sb = new SearchSourceBuilder();<br> sb.query(buildMainQuery(formObject));</p> <p>// Paging<br> sb.from(maxSize * formObject.getPage());<br> sb.size(maxSize);</p> <p>// Fields to return<br> sb.storedFields(asList(formObject.getRequestedFields()));</p> <p>// Filters<br> sb.postFilter(buildFilterQuery(formObject));</p> <p>// Sorting<br> sb.sort(formObject.getSortField(), toSortOrder(formObject.getSortOrder()));</p> <p>SearchRequest request = new SearchRequest(indexName);<br> request.types(objectType);<br> request.source(sb);</p> <p>(...)</p> <p>SearchResponse searchResponse = client.search(searchRequest);<br>
The query builders were accepted as they were, and the search response object was the same. We ran the tests, and they were still green. The search service no longer depended on the transport client. For indexing operations, the conversion was similarly straight forward:
<br> BulkRequestBuilder indexBulkRequestBuilder = client.prepareBulk();<br> (...)<br> IndexRequestBuilder indexRequestBuilder = client.prepareIndex(indexName, objectType);<br> indexRequestBuilder.setId(domainObject.getId());<br> String json = jsonConverter.convertToJson(domainObject);<br> indexRequestBuilder.setSource(new BytesArray(json), XContentType.JSON);<br> indexBulkRequestBuilder.add(indexRequestBuilder);<br> (...)<br> BulkResponse response = indexBulkRequestBuilder.execute().actionGet();<br>
And with the Low level REST client:
<br> BulkRequest bulkRequest = new BulkRequest();<br> (...)<br> IndexRequest indexRequest = new IndexRequest();<br> String json = jsonConverter.convertToJson(domainObject);<br> indexRequest.index(indexName);<br> indexRequest.id(domainObject.getId());<br> indexRequest.source(new BytesArray(json), XContentType.JSON);<br> indexRequest.type(objectType);<br> bulkRequest.add(indexRequest);<br> (...)<br> BulkResponse response = client.bulk(bulkRequest);<br>
Now for the admin operations that manage indices. In our target release of Elasticsearch, 6.0.2, the indices API was not yet supported. In the current release (6.2 at the time of writing this blog), there is support for this, so at first we tried using the 6.2 version of the ES libraries, against a cluster running 6.0.2. This turned out to break the search functionality. The 6.2 version of the query builders was including keywords in some queries, that were not yet supported in 6.0. Elastic had warned about this in their announcement mentioned earlier:
“… the high-level client still depends on Elasticsearch, like the Java API does today. This may not be ideal, as it still ties users of the client to depend on a certain version of Elasticsearch, but this decision allows users to migrate away more easily from the transport client. We would like to get rid of this direct dependency in the future, but since this is a separate long-term project, we didn’t want this to affect the timing of the client’s first release.”
As the High Level REST Client implementation wasn’t directly compatible with the admin operations in our application anyway, we decided to just implement these features using the Low Level REST client. Here is an example of creating an index template using the transport client
<br> String template = readFile(templateFileName);</p> <p>elasticClient.admin()<br> .indices()<br> .preparePutTemplate(indexName)<br> .setPatterns(indexNamePattern)<br> .setSource(new BytesArray(template), XContentType.JSON)<br> .get();<br>
And here is the same operation using the low level REST client:
<br> String template = readFile(templateFileName);</p> <p>HttpEntity entity = new NStringEntity(template, ContentType.APPLICATION_JSON);</p> <p>client.performRequest("PUT", "_template/" + templateName, emptyMap(), entity);<br>
The index name pattern now had to be included in the template JSON and was no longer dynamically configurable in the code, but this was not a problem for our application. With all the tests nice and green, the project was done!
Conclusion
Software problems always seem easy once you know how to solve them. This report from the trenches may give the impression that this was a simple, straight forward migration that only took a few days, but in reality the whole project involved several weeks of investigation, backtracking from dead ends, and a lot of trial and error. Hopefully, this blog can help fellow Java developers who are tasked with the same problem save a lot of time, or you could contact Trifork to provide a helping hand.
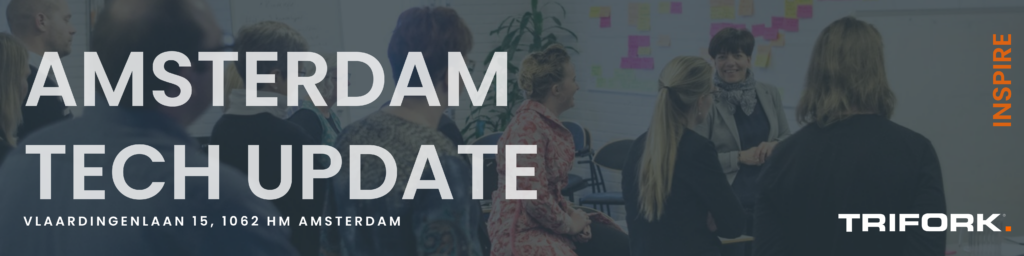