Spring Data Native Queries and Projections in Kotlin
This blog describes the solution to mapping native queries to objects. This is useful because sometimes you want to use a feature of the underlying database implementation (such as PostgreSQL) that is not part of the JPQL standard. By the end of this blog you should be able to confidently use native queries and use their outcome in a type-safe way.
In creating great applications based on Machine Learning solutions, we often come across uses for frameworks and databases that aren’t exactly standard. We sometimes need to build functionality that is either so new or so specific that it hasn’t been adopted into JPA implementations yet.
Working on a project with Spring Data is usually simple albeit somewhat opaque. Write a repository, annotate methods with @Query annotation and presto! You have mapped your database entities to Kotlin objects. Especially since Spring Framework 5 many of the interoperability issues (such as nullable values that are never null) have been alleviated.
Confucius wrote “Real knowledge is to know the extent of one’s ignorance”. So, to gauge the extent of our ignorance, let’s have a look at what happens when we cannot use the JPA abstraction layer in full and instead need to work with native queries.
Setting up the entity
When you use non-JPA features of the underlying database store, things can become complex.
Let’s say we have the following PostgreSQL table for storing people:
CREATE TABLE person ( id BIGSERIAL NOT NULL UNIQUE PRIMARY KEY, first_name VARCHAR(20), last_name VARCHAR(20) );
Given we represent an individual person like this:
import javax.persistence.Entity import javax.persistence.GeneratedValue import javax.persistence.Id import javax.persistence.Table
@Entity @Table(name = "person") class PersonEntity { @Id @GeneratedValue var id: Long? = null var firstName: String? = null var lastName: String? = null }
We can access that using a Repository:
import org.springframework.data.jpa.repository.JpaRepository import org.springframework.stereotype.Repository
@Repository interface PersonRepo : JpaRepository<PersonEntity, Long>
We could now implement a custom query on the repository as follows:
@Repository interface PersonRepo : JpaRepository<PersonEntity, Long> { @Query("FROM PersonEntity WHERE first_name = :firstName") fun findAllByFirstName(@Param("firstName") firstName: String): List<PersonEntity> }
So far so good. It uses JPQL syntax to form database-agnostic queries which is nice because we get some validation of these queries when starting the application, plus the added benefit of the syntax being database-type ignorant.
Adding a native query
Sometimes however, we want to use syntax that is specific to the database that we are using. We can do that by adding the boolean nativeQuery
attribute to the @Query
annotation and using Postgres’ SQL instead of JPQL:
@Query("SELECT first_name, random() AS luckyNumber FROM person", nativeQuery = true) fun getPersonsLuckyNumber(): LuckyNumberProjection?
Obviously this example is simple for the sake of this context, more practical applications are in the area of using the extra data types that Postgres offers such as the cube data type for storing matrices.
You may be, as I was at first, tempted to write a class for LuckyNumberProjection.
class LuckyNumberProjection { var firstName: String? = null var luckyNumber: Float? = null }
You will run cause into the following error:
org.springframework.core.convert.ConverterNotFoundException: No converter found capable of converting from type [org.springframework.data.jpa.repository.query.AbstractJpaQuery$TupleConverter$TupleBackedMap] to type [com.trifork.machinelearning.PersonRepo$LuckyNumberProjection]
The accompanying stack trace points in the direction of converters. This then makes you need to add a converter. However that doesn’t seem like it should be as hard. Good for us it turns out it isn’t!
Turns out that contrary to Entities, Projections, like Repositories, are expected to be interfaces. So let’s do that instead:
interface LuckyNumberProjection { val firstName: String? val luckyNumber: Float }
This should set you straight next time you want to get custom objects mapped out of your JPA queries.
At Trifork Amsterdam, we are currently doing multiple projects using Kotlin using frameworks such as Spring Boot, Axon Framework and Project Reactor on top of Kubernetes clusters using Helm to build small and smart microservices. More and more of those microservices contain our Machine Learning based solutions. These are in a variety of areas ranging from natural language processing (NLP) to time-series analysis and clustering data for recommender systems and predictive monitoring.
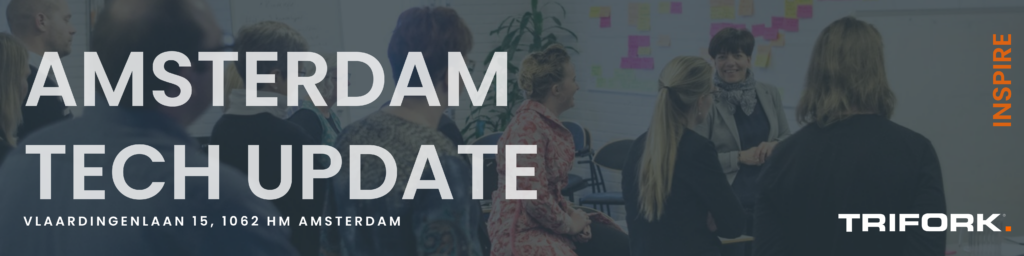