Ansible – Example playbook to setup Jenkins slave
As mentioned in my previous post about Ansible, we will now proceed with writing an Ansible playbook. Playbooks are files containing instructions that can be processed by Ansible, they are written in yaml. For this blog post I will show you how to create a playbook that will setup a remote computer as a Jenkins slave.
What do we need?
We need some components to get a computer execute Jenkins jobs:
- JVM 7
- A dedicated user that will run the Jenkins agent
- Subversion
- Maven (with our configuation)
- Jenkins Swarm Plugin and Client
Why Jenkins Swarm Plugin
We use the Swarm Plugin, because it allows a slave to auto-discover a master and join it automatically. We hence don’t need any actions on the master.
JDK7
We now proceed with adding the JDK7 installation task. We will not use any package version (for example dedicate Ubuntu PPA or RedHat/Fedora repos), we will use the JDK7 archive from oracle.com.
There multiple steps required:
- We need wget to be install. This is needed to download the JDK
- To download the JDK you need to accept terms, we can’t do that in a batch run so we need to wrap a wget call in a shell script to send extra HTTP headers
- Set the platform wide JDK links (java and jar executable)
Install wget
We want to verify that wget is installed on the remote computer and if not install it from the distribution repos. To install packages, there are modules available, yum and apt (There are others but we will focus on these).
To be able to run the correct task depending on the ansible_pkg_mgr value we can use only_id:
<br> - name: Install wget package (Debian based)<br> action: apt pkg='wget' state=installed<br> only_if: "'$ansible_pkg_mgr' == 'apt'"</p> <p> - name: Install wget package (RedHat based)<br> action: yum name='wget' state=installed<br> only_if: "'$ansible_pkg_mgr' == 'yum'"<br>
Download JDK7
To download JDK7 from oracle.com, we need to accept the terms but we can’t do that in a batch, so we need to skip that:
Create a script contains the wget call:
<br> #!/bin/bash</p> <p>wget --no-cookies --header "Cookie: gpw_e24=http%3A%2F%2Fwww.oracle.com" http://download.oracle.com/otn-pub/java/jdk/7/$1 -O $1<br>
The parameter is the archive name.
<br> - name: Copy download JDK7 script<br> copy: src=files/download-jdk7.sh dest=/tmp mode=0555</p> <p> - name: Download JDK7 (Ubuntu)<br> action: command creates=${jvm_folder}/jdk1.7.0 chdir=${jvm_folder} /tmp/download-jdk7.sh $jdk_archive<br>
These two tasks copy the script to /tmp and then execute it. $jdk_archive is a variable containing the archive name, it can be different depending on the distribution and the architecture.
Ansible provide a way to load variable files:
<br> vars_files:</p> <p> - [ "vars/defaults.yml" ]<br> - [ "vars/$ansible_distribution-$ansible_architecture.yml", "vars/$ansible_distribution.yml" ]<br>
This will load the file vars/defauts.yml (Note that all these file are written in yaml) and then look for the file vars/$ansible_distribution-$ansible_architecture.yml.
The variables are replaced by the their value on the remote computer voor example on an Ubuntu 32bits on i386 distribution, Ansible will look for the file vars/Ubuntu-i386.yml. If it doesn’t find it, it will fallback to vars/Ubuntu.yml.
Examples, Ubuntu-i386.yml would contain:
<br> ---<br> jdk_archive: jdk-7-linux-i586.tar.gz<br>
Fedora-i686.yml would contain:
<br> ---<br> jdk_archive: jdk-7-linux-i586.rpm<br>
Unpack/Install JDK
You notice that for Ubuntu we use the tar.gz archive but for Fedora we use an rpm archive. That means the the installation of the JDK will be different depending on the distribution.
<br> - name: Unpack JDK7<br> action: command creates=${jvm_folder}/jdk1.7.0 chdir=${jvm_folder} tar zxvf ${jvm_folder}/$jdk_archive --owner=root<br> register: jdk_installed<br> only_if: "'$ansible_pkg_mgr' == 'apt'"</p> <p> - name: Install JDK7 RPM package<br> action: command creates=${jvm_folder}/latest chdir=${jvm_folder} rpm --force -Uvh ${jvm_folder}/$jdk_archive<br> register: jdk_installed<br> only_if: "'$ansible_pkg_mgr' == 'yum'"<br>
On ubuntu, we just unpack the downloaded archive but for fedora we install it using rpm.
You might want to review the condition (only_if) particularly if you use SuSE.
jvm_folder is just an extra variable that can be global of per distribution, you need to place if in a vars file.
Note that the command module take a ‘creates’ parameter. It is useful if you don’t want to rerun the command, the module that the file or directory provided via this parameter exits, if it does it will skip that task.
In this task, we use register. With register you can store the result of a task into a variable (in this case we called it jdk_installed).
Set links
To be able to make the java and jar executables accessible to anybody (particularly our jenkins user) from anywhere, we set symbolic links (actually we just install an alternative).
<br> - name: Set java link<br> action: command update-alternatives --install /usr/bin/java java ${jvm_folder}/jdk1.7.0/bin/java 1<br> only_if: '${jdk_installed.changed}'</p> <p> - name: Set jar link<br> action: command update-alternatives --install /usr/bin/jar jar ${jvm_folder}/jdk1.7.0/bin/jar 1<br> only_if: '${jdk_installed.changed}'<br>
Here we reuse the stored register, jdk_installed. We can access the changed attribute, if the unpacking/installation of the JDK did do something then changed will be true and the update-alternatives command will be ran.
Cleanup
To keep things clean, you can remove the downloaded archive using the file module.
<br> - name: Remove JDK7 archive<br> file: path=${jvm_folder}/$jdk_archive state=absent<br>
We are done with the JDK.
Obviously you might want to reuse this process in other playbooks. Ansible let you do that.
Just create a file with all this task and include it in a playbook.
<br> - include: tasks/jdk7-tasks.yml jvm_folder=${jvm_folder} jdk_archive=${jdk_archive}<br>
jenkins user
Creation
With the name module, the can easily handle users.
<br> - name: Create jenkins user<br> user: name=jenkins comment="Jenkins slave user" home=${jenkins_home} shell=/bin/bash<br>
The variable jenkins_home can be defined in one of the vars files.
Password less from Jenkins master
We first create the .ssh in the jenkins home directory with the correct rights. And then with the authorized_key module, we can add the public of the jenkins user on the jenkins master to the authorized keys of the jenkins user (on the new slave). And then we verify that the new authorized_keys file has the correct rights.
<br> - name: Create .ssh folder<br> file: path=${jenkins_home}/.ssh state=directory mode=0700 owner=jenkins</p> <p> - name: Add passwordless connection for jenkins<br> authorized_key: user=jenkins key="xxxxxxxxxxxxxx jenkins@master"</p> <p> - name: Update authorized_keys rights<br> file: path=${jenkins_home}/.ssh/authorized_keys state=file mode=0600 owner=jenkins<br>
If you want jenkins to execute any command as sudo without the need of providing a password (basically updating /etc/sudoers), the module lineinfile can do that for you.
That module checks ‘regexp’ against ‘dest’, if it matches it doesn’t do anything if not, it adds ‘line’ to ‘dest’.
<br> - name: Tomcat can run any command with no password<br> lineinfile: "line='tomcat ALL=NOPASSWD: ALL' dest=/etc/sudoers regexp='^tomcat'"<br>
Subversion
This one is straight forward.
<br> - name: Install subversion package (Debian based)<br> action: apt pkg='subversion' state=installed<br> only_if: "'$ansible_pkg_mgr' == 'apt'"</p> <p> - name: Install subversion package (RedHat based)<br> action: yum name='subversion' state=installed<br> only_if: "'$ansible_pkg_mgr' == 'yum'"<br>
Maven
We will put maven under /opt so we first need to create that directory.
<br> - name: Create /opt directory<br> file: path=/opt state=directory<br>
We then download the maven3 archive, this time it is more simple, we can directly use the get_url module.
<br> - name: Download Maven3<br> get_url: dest=/opt/maven3.tar.gz url=http://apache.proserve.nl/maven/maven-3/3.0.4/binaries/apache-maven-3.0.4-bin.tar.gz<br>
We can then unpack the archive and create a symbolic link to the maven location.
<br> - name: Unpack Maven3<br> action: command creates=/opt/maven chdir=/opt tar zxvf /opt/maven3.tar.gz</p> <p> - name: Create Maven3 directory link<br> file: path=/opt/maven src=/opt/apache-maven-3.0.4 state=link<br>
We use again update-alternatives to make mvn accessible platform wide.
<br> - name: Set mvn link<br> action: command update-alternatives --install /usr/bin/mvn mvn /opt/maven/bin/mvn 1<br>
We put in place out settings.xml by creating the .m2 directory on the remote computer and copying a settings.xml (we backup any already existing settings.xml).
<br> - name: Create .m2 folder<br> file: path=${jenkins_home}/.m2 state=directory owner=jenkins</p> <p> - name: Copy maven configuration<br> copy: src=files/settings.xml dest=${jenkins_home}/.m2/ backup=yes<br>
Clean things up.
<br> - name: Remove Maven3 archive<br> file: path=/opt/maven3.tar.gz state=absent<br>
Swarm client
You first need to install the Swarm plugin as mentioned here.
Then you can proceed with the client installation.
First create the jenkins slave working directory.
<br> - name: Create Jenkins slave directory<br> file: path=${jenkins_home}/jenkins-slave state=directory owner=jenkins<br>
Download the Swarm Client.
<br> - name: Download Jenkins Swarm Client<br> get_url: dest=${jenkins_home}/swarm-client-1.8-jar-with-dependencies.jar url=http://maven.jenkins-ci.org/content/repositories/releases/org/jenkins-ci/plugins/swarm-client/1.8/swarm-client-1.8-jar-with-dependencies.jar owner=jenkins<br>
When you start the swarm client, it will connect to the master and the master will automatically create a new node for it.
There are a couple of parameters to start the client. You still need to provided a login/password in order to authenticate. You obviously want this information to be parameterizable.
First we need a script/configuration to start the swarm client at boot time (systemv, upstart or systemd it is up to you). In that script/configuration, you need to add the swarm client run command:
<br> java -jar {{jenkins_home}}/swarm-client-1.8-jar-with-dependencies.jar -name {{jenkins_slave_name}} -password {{jenkins_password}} -username {{jenkins_username}} -fsroot {{jenkins_home}}/jenkins-slave -master https://jenkins.trifork.nl -disableSslVerification &> {{jenkins_home}}/swarm-client.log &<br>
Then using the template module, to process the script/configuration template (using Jinja2) into a file that will be put on a given location.
<br> - name: Install swarm client script<br> template: src=templates/jenkins-swarm-client.tmpl dest=/etc/init.d/jenkins-swarm-client mode=0700<br>
The file mode is 700 because we have a login/password in that file, we don’t want people (that can log on the remote computer) to be able to see that.
Instead of putting jenkins_username and jenkins_password in vars files, you can prompt for them.
<br> vars_prompt:</p> <p> - name: jenkins_username<br> prompt: "What is your jenkins user?"<br> private: no<br> - name: jenkins_password<br> prompt: "What is your jenkins password?"<br> private: yes<br>
And then you can verify that they have been set.
<br> - fail: msg="Missing parameters!"<br> when_string: $jenkins_username == '' or $jenkins_password == ''<br>
You can now start the swarm client using the service module and enable it to start at boot time.
<br> - name: Start Jenkins swarm client<br> action: service name=jenkins-swarm-client state=started enabled=yes<br>
Run it!
<br> ansible-playbook jenkins.yml --extra-vars "host=myhost user=myuser" --ask-sudo-pass<br>
By passing ‘–ask-sudo-pass’, you tell Ansible that ‘myuser’ requires a password to be typed in order to be able to run the tasks in the playbook.
‘–extra-vars’ will pass on a list of viriables to the playbook. The begining of the playbook will look like this:
<br> ---</p> <p>- hosts: $host<br> user: $user<br> sudo: yes</p> <p>
‘sudo: yes’ tells Ansible to run all tasks as root but it acquires the privileges via sudo.
You can also use ‘sudo_user: admin’, if you want Ansible to run the command to sudo to admin instead of root.
Note that if you don’t need facts, you can add ‘gather_facts: no’, this will spend up the playbook execution but that requires that you know everything you need about the remote computer.
Conclusion
The playbook is ready. You can now easily add new nodes for new Jenkins slaves thanks to Ansible.
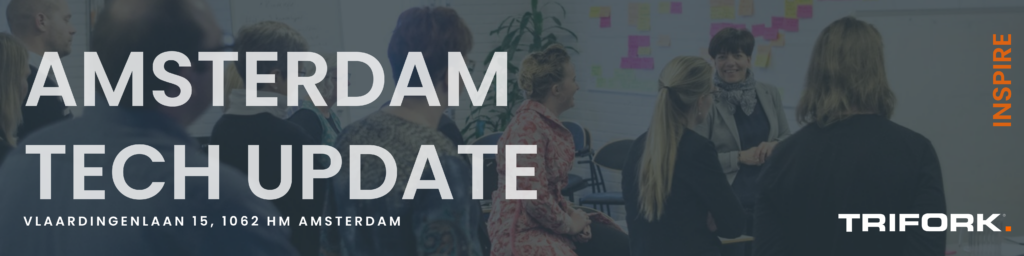